Digital Production, Design and Development
Task 2
Developing the Solution
This tutorial will guide you through how to add functionality to a Bootstrap HTML/CSS template. It is based on the Bean and Brew example project from Pearson, which you can download here.
For this project, you will need to download the Bootstrap template (click on the image opposite). You will also need server software (I recommend Xampp), and you will need a code editor (I recommend VS Code with the Devsense PHP extension). We will be developing the solution using PHP and MySQL (these are installed with Xampp).
Developing the Solution: Why PHP?
PHP - Hypertext Preprocessor (PHP used to stand for Personal Home Page, which would make more sense!) - is a server-side scripting language for web development. It has been around pretty much since the dawn of web development time, first released in 1995. The current version is 8.4.2 (as of December 2024) and it is constantly being updated. For the purposes of this project, we'll be using version 8.2.12 which is shipped with Xampp.
Despite it's age, PHP is still going strong - according to Wikipedia it is used on over 75% of websites. It is also the language of WordPress, Drupal, Joomla and Moodle. PHP is easy to learn (especially if you have already done Python). It also covers concepts such as arrays, do...while loops, constants and superglobals which are not available in Python.
Learning PHP as your first server-side development language will give you a good foundation to move on to other languages such as Ruby, Java and C# later on.
You are allowed to access reference resources for Task 2. I would recommend W3Schools, which provides useful tutorials.
Bootstrap
Bootstrap is a front-end CSS framework, originally developed by Twitter in 2011. It is free and open-source and you can download many HTML/CSS templates which use the Bootstrap framework which will help you get started with your project. Using templates will save you a lot of development time which you can use to concentrate on developing the most important aspect of the task - the back-end functionality.
You can do a Google search for Bootstrap templates. This counts as an asset so you need to record where you find a template you want to use. Bootstrap is currently in version 5, but some templates use version 4. There are some differences between the two. You can find out which version your template uses by viewing the bootstrap.min.css file (usually found in the CSS folder). The template we will be using uses version 4.
MySQL
SQL (Structured Query Language) is a database language. It allows you to create, read, update, and delete data in a database. The database we'll be using is MariaDB, this is a fork of MySQL which comes bundled with XAMPP. It supports all MySQL functions.
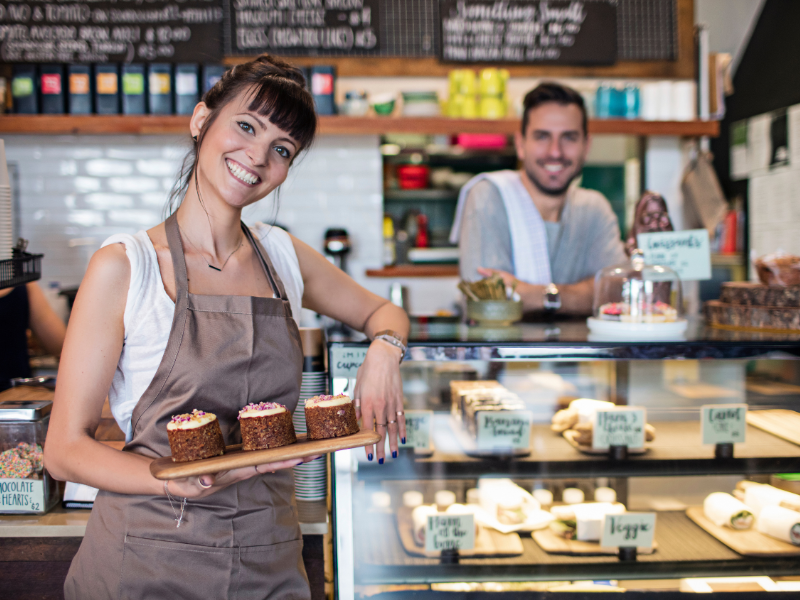
Bean and Brew
For this project, you need to develop a digital solution for a chain of coffee shops called Bean and Brew (a fictional company based in Yorkshire). They want to create a solution to allow their customers to book a table, order products for collection, book baking lessons, and create accounts for a personalised experience.
To meet the needs of this client you need to build a website which will allow customers to:
Requirement 1: Book a table at one of the restaurants.
Requirement 2: Order coffee and bakery products for collection
Requirement 3: Create an online account
Requirement 4: Book an online bakery class
Requirement 5: Create customer bakery hampers
Required Resources
Project Brief (pdf)
Introduction to Task 2 (pdf)
Content Assets Log (doc)
Test Log (doc)
You will need to unzip and save the Bootstrap template in the /htdocs folder in C:\xampp. You also need to start the Apache and MySQL services using the XAMPP control panel to be able to view files you create using PHP code. You can view php files in a web browser (such as Chrome) using the URL: localhost/coffeeshop/index.php
Do this first!
How to Convert a Bootstrap HTML Template to a PHP Website Video Tutorial (no sound)
Bean and Brew Solution Version 1
MODULE 1: TABLE BOOKING
Requirement 1: Book a space at one of the 3 local restaurants (Harrogate, Leeds, Knaresborough Castle).
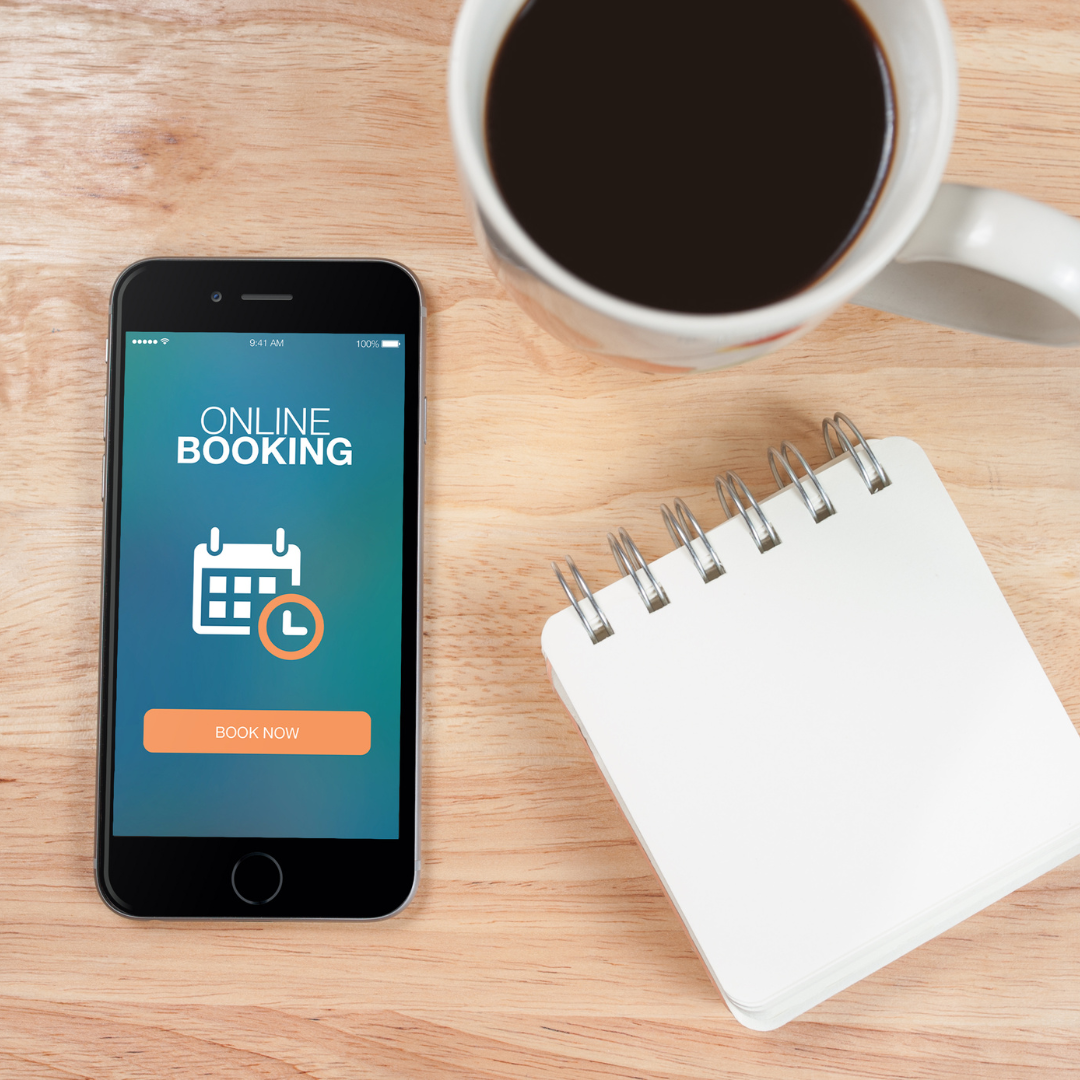
MODULE 1 Resources
Bookings Table SQL (txt)
Database Connection Code (txt)
Basic HTML Booking Form (txt)
Blank Page Template (txt)
Video Tutorial 1: How to Code a Booking Form (YouTube)
MODULE 2 ONLINE ORDERING
Requirement 2: Be able to pre-order coffee and baked goods for collection.
MODULE 2 RESOURCES
Products Table SQL (txt)
Sample Product Data (txt) Generated by CoPilot
Cart Table SQL (txt)
Product Images (zip) From Canva
Check Cart Function (php)
Sample Discounts (txt)
Discount Box (txt)
Revised Order Form (txt)
Orders Table SQL (txt)
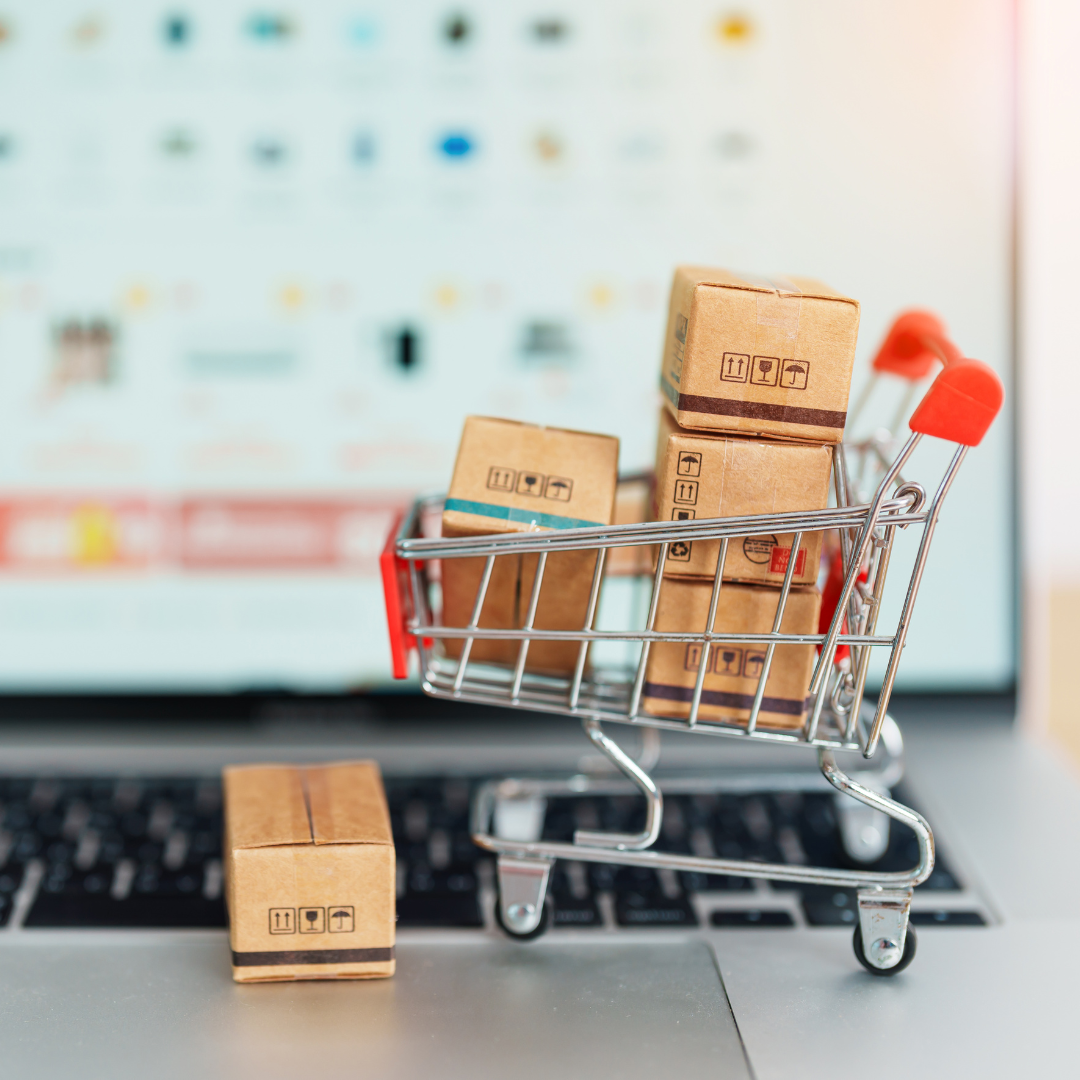
MODULE 2 CODING TUTORIALS
1: Displaying Products
Video Tutorial 2 - Displaying Products (YouTube)
2: Adding Products to the Cart
3: Updating and Removing Products
4: Calculating Totals and Applying Discounts
5: Ordering & Checkout
Video Tutorial 6 - Ordering & Checkout (YouTube)
6: Fix the Cart
Video Tutorial 7 - Fixing the Cart (YouTube)
MODULE 3 CUSTOMER ACCOUNTS
Requirement 3: Creating customer accounts to speed up re-ordering
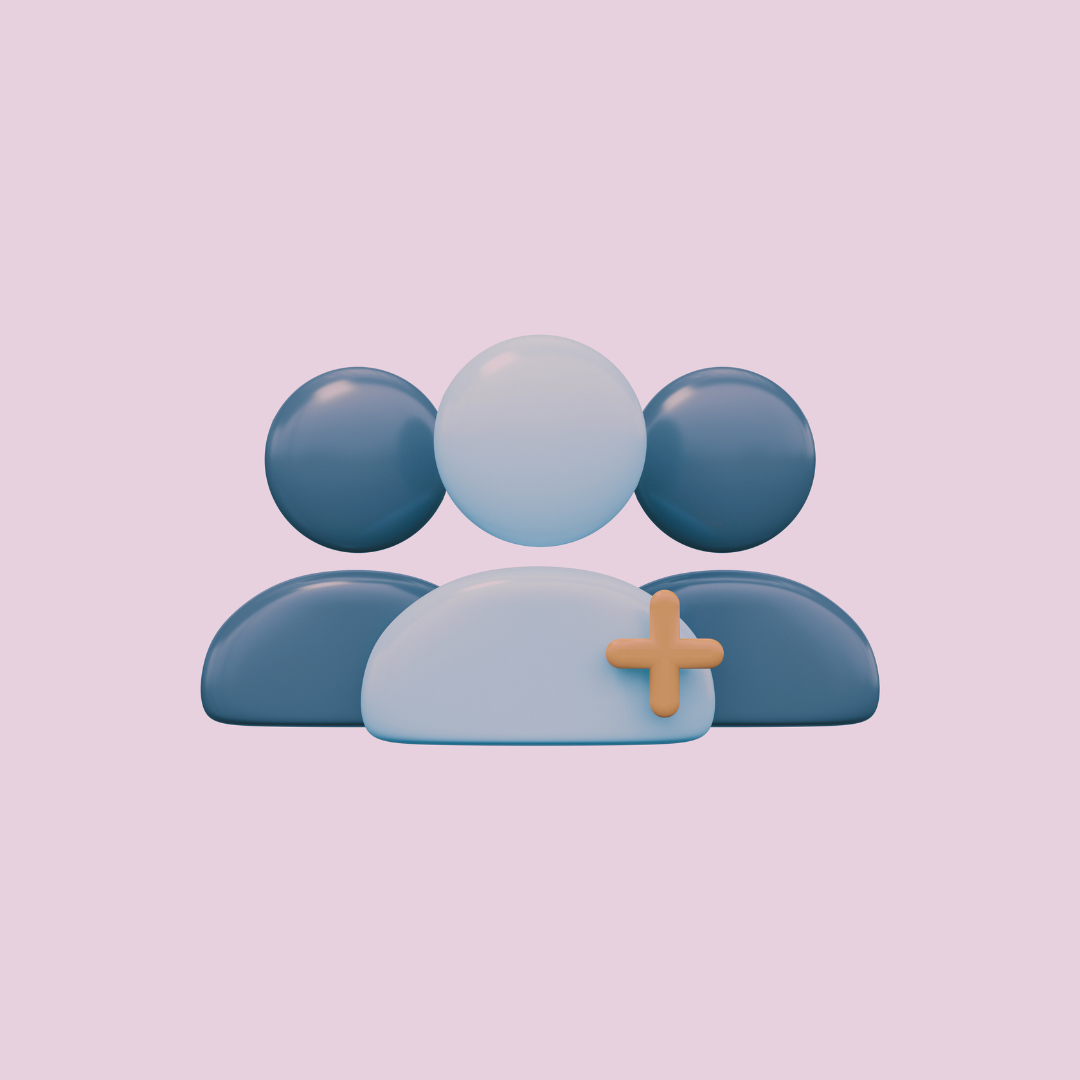
MODULE 3 RESOURCES
Users Table (txt)
Register User Code (txt)
Logout Script (php)
Video Tutorial 8 - User Accounts (YouTube)
MODULE 4 EVENT BOOKING
Requirement 4: Allow customers to pre-book (online) baking lessons.
MODULE 4 RESOURCES
Events Table (txt)
Events Sample Data (txt) Generated by CoPilot
Registrations Table (txt)
Events Images (zip) From Canva
Events Page Template (txt)
Video Tutorial 9 - Creating an Event Booking Form (YouTube) - NO SOUND
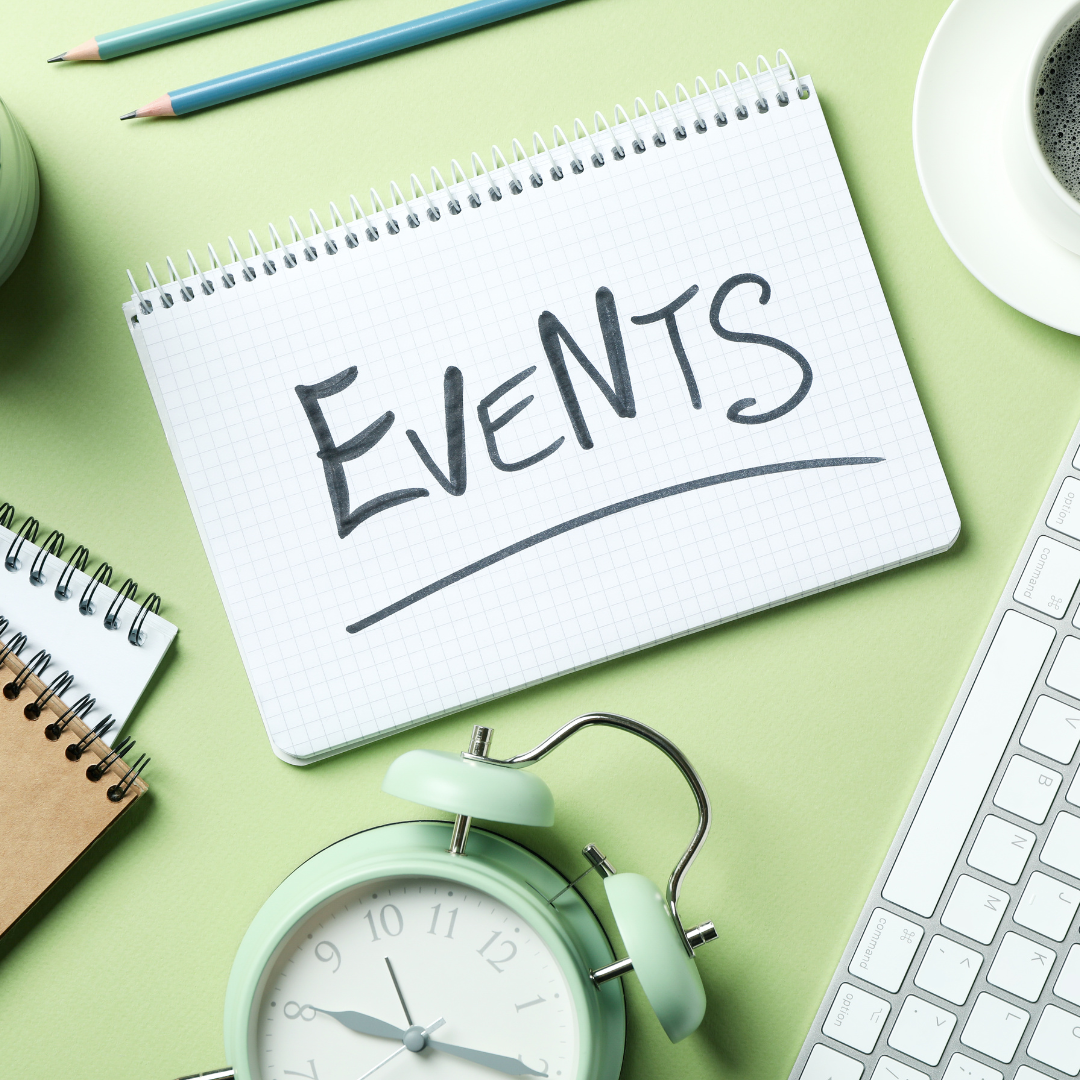
MODULE 5 CUSTOM HAMPERS
Requirement 5: Allow customers to create custom baked goods hampers.
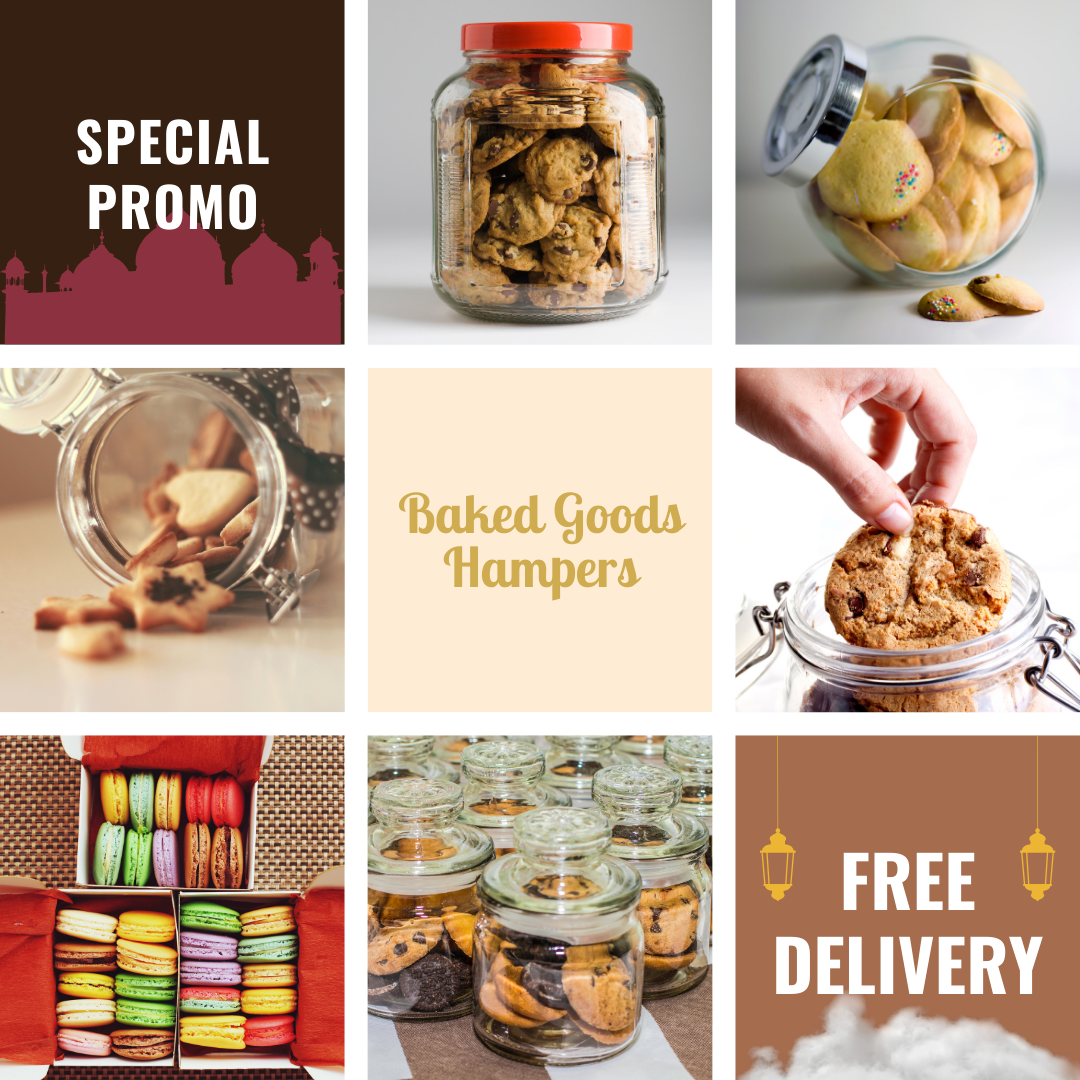
MODULE 5 RESOURCES
Gift Hamper Image (png) - save this in your products folder
Hamper Product Images (zip)
Hampers Table (txt)
Hampers Sample Data (txt)
Delivery Table (txt)
Video Tutorial 10 - Creating Custom Products (Coming Soon)
Branding the Prototype
A prototype is much more impressive for clients when it's personalised to their brand. This video will show you how to change the front-end so that it is more personal to the Bean and Brew brand.
Some text about Bean and Brew:
We were one of the first companies in the UK to use fair-trade coffee and all organic milk for our products. Each drink is made exactly to your specifications, for a personal service.
Add this call to Share This API in header.php
<script type="text/javascript" src="https://platform-api.sharethis.com/js/sharethis.js#property=678d3b47c7696f0012589a28&product=inline-share-buttons&source=platform" async="async"></script>
Video Tutorial 11 - Branding the Prototype (Coming Soon)
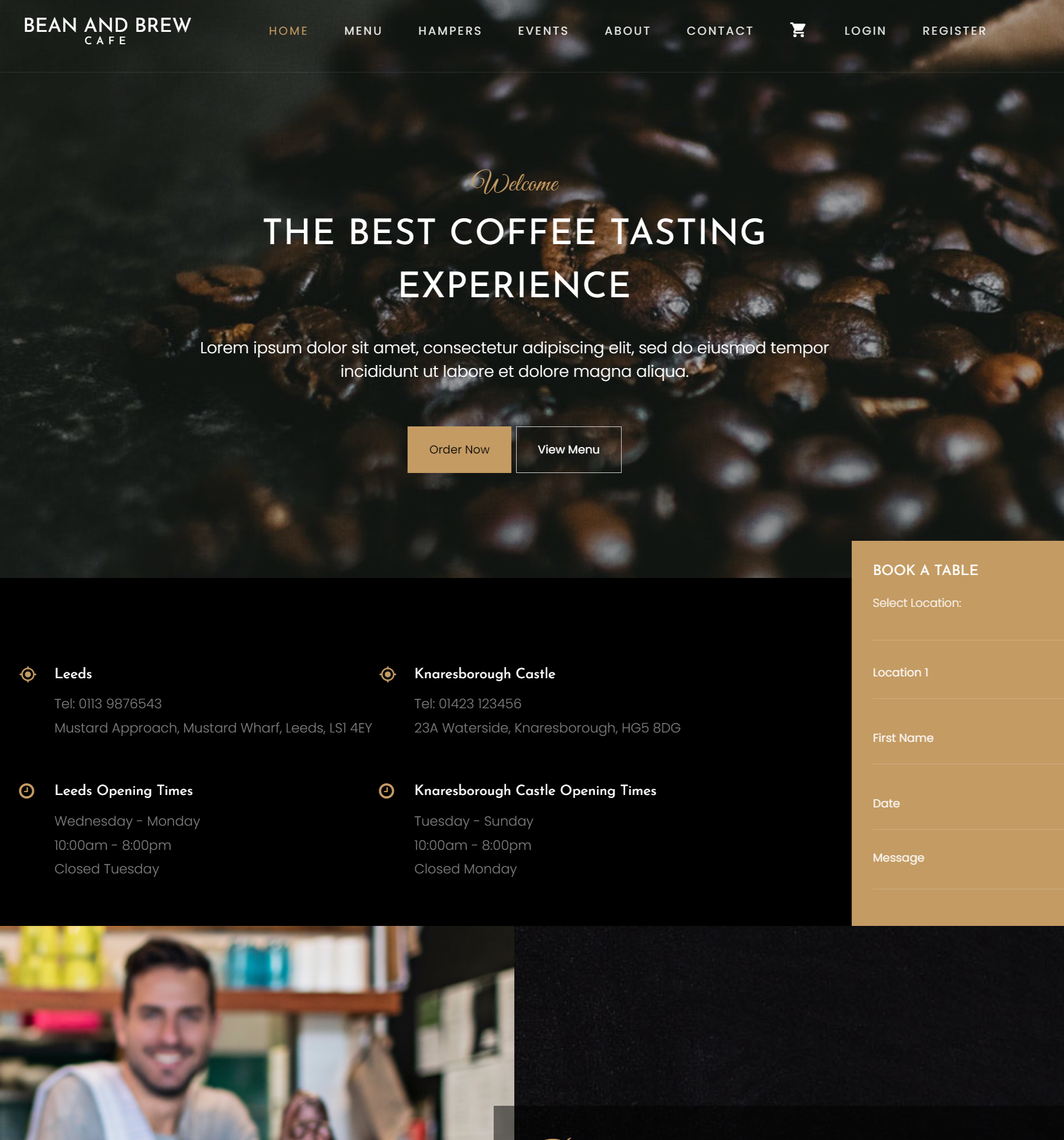
Problems?
If you're having trouble with discounts not applying or anything stored in session variables then try this:
1. In the xampp folder you will find a folder called tmp
2. Delete all the text files that start with sess_ This is where the data for the superglobal session variables are stored - you can also open these to check the contents of session variables if you need to.
3. Restart Apache and open a new browser tab (to make sure the session id is reset)